Front-end frameworks like Angular enable developers to build scalable applications that can run on multiple device types - for web, mobile web, native mobile and native desktop. TinyMCE is Angular-friendly product that offers reliable Angular rich text editor solution.
Frequent updates to Angular can leave developers in a bit of a pickle when trying to decide which feature to use and how to use them correctly. So to maintain the high quality of your applications, there are certain things you should look out for when using Angular. This article discusses some of the common mistakes and how to avoid them.
1. Failing to unsubscribe at the right time
When using JavaScript, we often subscribe to events or observables. However, many developers either forget to unsubscribe when the intended activity is finished, or even ignore it out of carelessness. Lingering subscriptions can cause memory leaks and lead to critical problems for your apps.
The two recommended practices to unsubscribe are:
- Initiate the OnDestroy lifecycle if it is present in the event you have subscribed to.
- Initiate the lifecycle yourself if the component does not have one.
import {HttpClient} from '@angular/common/http';
@Component({})
export class AppComponent implements OnInit, OnDestroy {
private subscription: Subscription;
constructor(private http: HttpClient) {
}
ngOnInit () {
subscription = this.http.get('/url').subscribe(() => { });
}
ngOnDestroy() {
this.subscription.unsubscribe();
}
}
2. Manipulating the DOM directly
You may come across situations where you have to manipulate the DOM directly. Angular provides high-level APIs for this purpose.
Some developers tend to use “ElementRef”, but this is not recommended. The Angular docs recommend using “ElementRef” as a last resort to access the DOM directly as it can expose applications and make them vulnerable to XSS attacks. It may also lead to tight coupling among the application and rendering layers.
@Component({ ... })
export class BasicComponent {
constructor(private _elementRef: ElementRef) {}
wrong() {
$('.wrong-way').click();
this._elementRef.nativeElement.xyz = ' ';
document.getElementById(' ');
}
}
To avoid the complications mentioned above, use Renderer2 instead of ElementRef. The Renderer API provides safe access and decouples the code from the browser, which in turn allows the application to run in web workers.
@Component({ ... })
export class BasicComponent {
constructor(private _renderer2: Renderer2,
private _elementRef: ElementRef) {}
right() {
this._renderer2.setProperty(this._elementRef, 'a_property', true);
}
}
3. Multiple component declarations
Components are the fundamental building blocks of all Angular applications. Every component should belong to an NgModule so that it is accessible by other components and views. You can make a component a member of the NgModule, by listing it in the @NgModule.declarations array.
The angular compiler does not allow you to declare the same component in different modules. However, there may be situations where you want to declare a similar component in another module. In this case, we have to indicate the relationship between similar modules - whether it’s a parent-child module connection or not - to avoid errors thrown by the Angular compiler.
The following example demonstrates how you can accomplish multiple declarations:
NgModule({
declarations: [BasicComponent],
exports: [BasicComponent]
}
export class Child { ... }
@NgModule({
imports: [Child_Module]
}
export class Parent { ... }
4. Use of jQuery with Angular apps
Although Angular and jQuery share a common foundation - JavaScript - they were built with different purposes in mind. Angular consists of many useful features, but developers often switch back to jQuery for a simple approach to DOM manipulation. If you do this, however, Angular won't know about how the DOM was manipulated by jQuery, thus you may experience unwanted effects. So it’s important that you really know what you’re doing before you take a mix and match approach with Angular and jQuery.
5. Misconception about ngOnChanges
ngOnChanges is a lifecycle hook in Angular to watch inputs to various components and trigger an action when the inputs are modified. However, this feature is not effective for every use case and has limitations. It only supports one-way use, which means ngOnChanges fires only when the whole object changes, but not when a property or field update occurs.
Some Angular users are not familiar with this concept, and this misunderstanding leads them to use ngOnChanges with unexpected outcomes. In cases where you need notifications for every update in a field, ngDoCheck is far more effective. However, ngDoCheck runs quite frequently, so you need to consider exactly what it’s executing. There are also alternative solutions, such as using subscriptions.
What next?
The examples listed here are among the most common mistakes developers make when using Angular, especially when they’re new to it, and they are easily avoided. This will not only improve application performance but also ensure a better user experience.
Angular is a versatile front-end framework that continues to mature while gaining more popularity and momentum. For more information about using Angular, you should check out the official Angular documentation.
While you’re here, also check out how to create an Angular reactive form with a rich text editor.
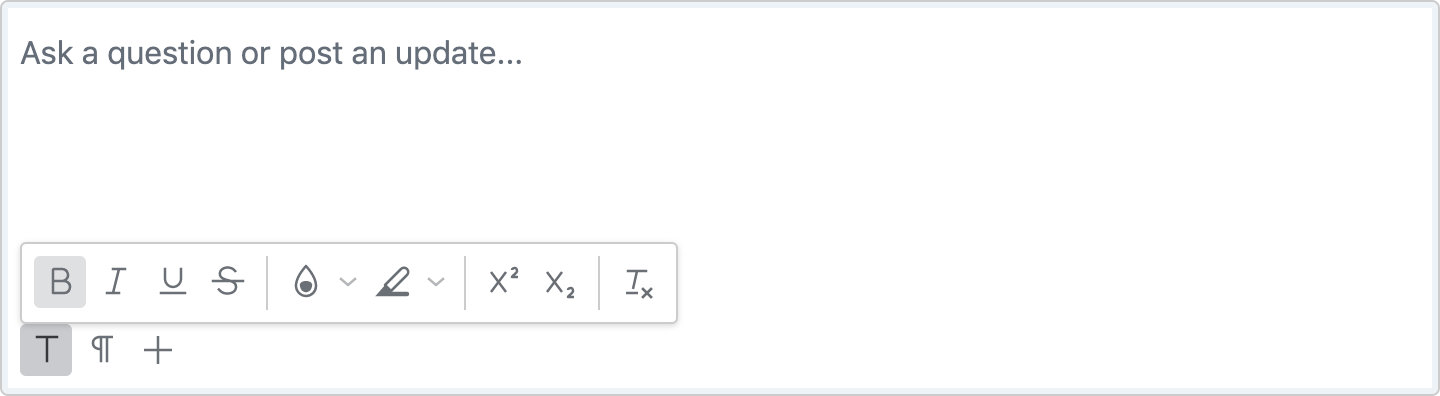