Your app needs a cloud service provider and a database. It's unavoidable. But sometimes, you need to let your customers save content without involving the database or cloud service provider. In this case, you can use JavaScript to access localStorage iny our browser. But you probably have questions: what is localStorage, when can you use it, and is it secure?
If you've already learned about localStorage, and you know what you're getting yourself into, check through the table of contents, or start with the JavaScript local storage demo. The demo makes use of the TinyMCE rich text editor as an example of a useful content builder.
Otherwise, read on to find out more about JavaScript and localStorage, what it is, and how it works.
Contents
What is localStorage in JavaScript
Advantages and limitations of localStorage
localStorage vs sessionStorage vs Cookie
SessionStorage vs localStorage
localStorage vs Cookie
IndexedDB vs localStorage
How to use localStorage methods in JavaScript
How to clear localStorage
localStorage JavaScript example
localStorage next steps
What is localStorage in JavaScript
localStorage in JavaScript allows web applications to store data locally within the user's browser – with no expiration date. The data isn’t deleted when the browser is closed, and is available when the browser is opened again.
Data stored remains saved depending on if the browser is using a best-effort method, or a persistent method. When best-effort is in effect, the data persists as long as the stored data does note exceed the limits, and customers don't manually delete the localStorage saved data. When persistent is used, data is only deleted, or evicted, by the customer's choice.
All browsers support localStorage through the api.Window.localStorage method, and you can log any browser compatibility issues at the MDN browser compatibility GitHub repository.
localStorage also allows your customers to access specific data quickly without the overhead of a database. There are other advantages and disadvantages to understand when working with localStorage.
✏️NOTE: Other options for data storage are available, like Uploadcare for example.
Advantages and disadvantages limitations of JavaScript localStorage
Advantages
- It stores up to 5MiB of data in local storage
- A maximum of 10MiB of data across bot localStorage and sessionStorage (data in sessionStorage is cleared when the page session ends)
- Easy to use – no need for a web server or backend database
- Great for getting an idea up and running and for testing purposes
Limitations
- It can only store strings, specifically UTF-16 string format, with two bytes per character. This can restrict you from using it for more complex scenarios although, HTML can be stored as a string
- It’s local to the browser and specific to the origin (per domain and protocol), and there’s no guarantee that the data will persist even in that same context
Security limitation
- It’s not secure. Don’t store any private or personal information in localStorage
localStorage vs sessionStorage vs cookie
The main difference between localStorage, sessionStorage, and cookie storage is size.
There’s:
- 10MiB available for localStorage
- 5MiB for sessionStorage
- 4KB for Cookies.
Of these three types of storage, localStorage is the newest kind of in-browser storage.
Unlike sessionStorage, data stored in localStorage persists, and is accessible even after the browser closes. On Stack Overflow, cssyphus put together a useful explanation that contrasts the different storage types along with a list of resources.
SessionStorage is useful when you need browser storage that does not impact web application performance. Login credentials are held in sessionStorage as they are cleared once the open tab closes.
Cookies are the oldest kind of in-browser storage. With their small capacity, they can hold small amounts of data, and are not designed to hold sensitive data such as login credentials.
Sessionstorage vs localStorage
Together, these two kinds of storage are the main data storage objects available that the browser can access easily for storing and retrieving information for customers. The main difference between Session and Local kinds of storage is the lifespan of the stored data.
SessionStorage is tied to a specific tab the browser may have open, whereas localStorage is accessible across any tab the browser has open. Once the tab closes, the sessionStorage is deleted, but localStorage persists.
localStorage vs cookie
Compared to localStorage, Cookies are older, and can only fit small amounts of data. There are two kinds of Cookies: session, and persistent.
- Session Cookies, like sessionStorage, expire when the browser closes.
- A persistent Cookie has an expiry attribute, or a max-age attribute that designates when the browser deletes the data held in cookie storage.
Cookies are accessible by servers, as when browsers interact with a server, cookie data is sent along with requests and responses.
IndexedDB vs local storage
A new kind of browser storage, IndexedDB appeared in an attempt to make an improved version of localStorage. Contrasting the two, IndexedDB has a more complex API syntax compared to localStorage. IndexedDB allows for the storage of structured data.
Unlike localStorage, IndexedDB is described as a JavaScript-based object-oriented database. Its design is to provide database-like behavior without the need for an internet connection to a web-server.
How to use localStorage methods in JavaScript
There are four basic JavaScript localStorage methods you can use to access and work with localStorage:
- setItem() - takes a key-value pair and adds it to localStorage
- getItem() - takes a key and returns the corresponding value
- removeItem() - takes a key and removes the corresponding key-value pair
- clear() - clears localStorage (for the domain)
To test out these methods, the following procedure uses the setItem() and getItem() methods. Feel free to try out the other methods in a similar way.
- Open a browser, and then access the console. For example, in Google Chrome, open Developer Tools and click on the Console tab.
- Enter the localStorage command, and the current stored data will be returned. For example (assuming it’s empty to begin with):
localStorage
Storage {length: 0}
- Enter localStorage.setItem('myKey', 'testValue') and the string testValue will be stored against the key myKey:
localStorage.setItem("myKey", "testValue");
undefined;
localStorage
Storage {myKey: "testValue", length: 1}
✏️NOTE: undefined just means that no value is returned from that method. Enter localStorage again to view the stored data.
- Enter localStorage.getItem('myKey') and the corresponding string will be returned:
localStorage.getItem("myKey");
("testValue");
✏️NOTE: Type localStorage. (with the ‘.’) into the console and a full list of relevant methods will appear.
How to clear localStorage
You can run the command clear() to completely clear the localStorage data (for the domain):
- Check if there is content stored in localStorage:
localStorage
Storage {length: 0}
- For the purpose of the test, add some content to clear:
localStorage.setItem("clearKey", "clearThisValue");
undefined;
- Clear the localStorage data using the clear() method:
localStorage.clear();
undefined;
- Check again to confirm the local storage is clear.
localStorage
Storage {length: 0}
localStorage JavaScript example
The following demo shows how you can store information from the TinyMCE text area in localStorage.
✏️NOTE:You can also take advantage of TinyMCE's Advanced features that connect with database storage to make your customer's content building process easier. For example, the TinyMCE Templates plugin is an Advanced plugin available with a TinyMCE paid plan. Templates and other Advanced plugins are available to try out with a 14-day FREE trial.
Create a simple index.html file with a single textarea, and two buttons as follows. The textarea id can be anything – in this example it's called myTextArea.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>TinyMCE LocalStorage</title>
<script src="https://cdn.tiny.cloud/1/ADD_YOUR_TINYMCE_API_KEY_HERE/tinymce/7/tinymce.min.js" referrerpolicy="origin"></script>
<script>
tinymce.init({
selector: "#myTextarea",
});
</script>
<style>
body {
padding: 10px;
}
</style>
</head>
<body>
<h1>JavaScript localStorage demo</h1>
<textarea id="myTextarea" rows="10" cols="80"></textarea>
<p></p>
<button onclick="saveContent()">Save</button>
<button onclick="loadContent()">Load</button>
<script>
function saveContent() {
var myContent = tinymce.activeEditor.getContent("myTextarea");
localStorage.setItem("myContent", myContent);
}
function loadContent() {
var myContent = localStorage.getItem("myContent");
tinymce.activeEditor.setContent(myContent);
}
</script>
</body>
</html>
On the ADD_YOUR_TINYMCE_API_KEY_HERE string: TinyMCE requires a valid API key, otherwise error messages concerning read-only mode can appear in the TinyMCE text area. Your API key is free, and you can access it on the TinyMCE dashboard using Google or GitHub credentials.
Get Your Free TinyMCE API Key →
The demo content has two JavaScript functions: saveContent() and loadContent(). The buttons are configured so that when clicked they trigger the relevant JavaScript function. Here’s how it works in more detail:
- The saveContent() function accesses the current value of the textarea and assigns it to a variable myContent
- getContent() retrieves the TinyMCE text area contents. You could also use document.getElementById() to access the textarea with id myTextArea
- localStorage.setItem() adds the retrieved editor content into localStorage
- The loadContent() function operates in a similar way, only in reverse
- localStorage.getItem() retrieves the saved data from localStorage
- setContent() then adds the retrieved data to the TinyMCE text area
Here's how the localStorage demo works with TinyMCE:
And the following image shows the demo with some custom CSS for the buttons to add more visual interest:
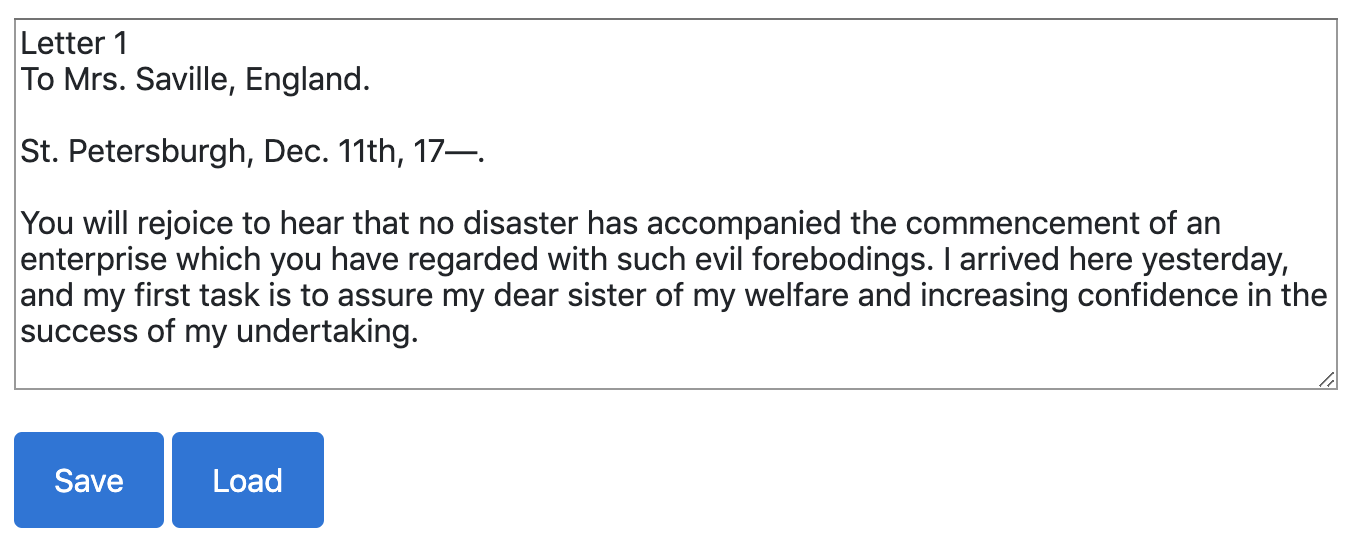
To test out the demo:
- Type something in the textarea and click Save. (For example, the demo contains a snippet of prose from Frankenstein) The content then saves to localStorage.
- Refresh the page, and the content will disappear.
- Click the Load button, and the content is retrieved from localStorage, and appears in the text area.
✏️NOTE: While you’re on the page, you could try opening the browser console from within Developer Tools and executing some of the localStorage methods as shown in the previous section to confirm what’s happening behind the scenes.
localStorage next steps
Why not try taking this example one step further? Check out the article with more information on adding a rich text editor that works with JavaScript localStorage:
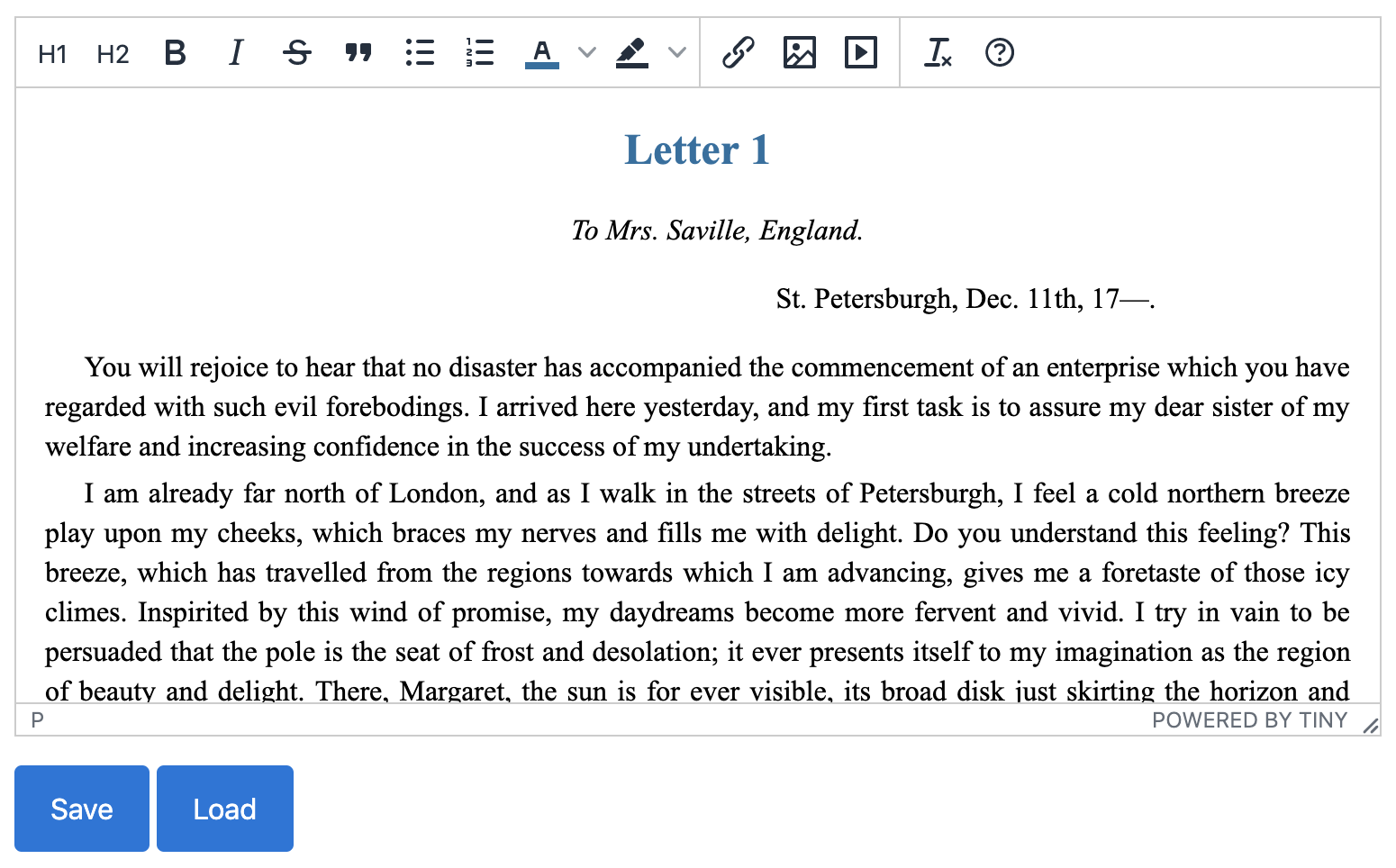
If you’d like to know more about Tiny, follow us on Twitter or send us a message if you have any questions on how your app could work with localStorage and TinyMCE.