Recently, I came across an awesome list of JavaScript snippets. It’s impressively exhaustive and helpful, but it got me wondering…what are the top 10 FAQs on "how to JavaScript"?
So, with no further ado, here they are (with answers)!
- How to add to an array
- How to call a function
- How to check for undefined
- How to convert string to number
- How to iterate through an object
- How to remove an item from an array
- How to check if an element exists
- How to redirect
- How to sleep
- How to print an object
BONUS TIP: How to add a JavaScript rich text editor to your project.
1. How to add to an array
Use the push()
method to add an item to a JavaScript array.
For example, in the following code, the value "Kiwi"
is added to the end of the fruits
array.
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.push("Kiwi");
console.log(fruits);
The push()
method can also be used to add multiple values to an array:
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.push("Kiwi", "Watermelon");
console.log(fruits);
Note, though, that this mutates the original array which goes against immutability practices. Here’s a great resource on how to work with arrays while practicing immutability.
2. How to call a function
Call a JavaScript function by referring to the name used to define it, including any expected parameters.
For example:
function square(number) {
return number * number;
}
console.log(square(5));
Also…
The JavaScript call()
method allows you to write a function that can be used on different objects.
For example:
var fruit = {
details: function () {
return this.color + " " + this.name;
},
};
var apple = {
name: "Apple",
color: "Red",
};
console.log(fruit.details.call(apple));
Check out the apply()
method too.
3. How to check for undefined
A JavaScript variable is undefined
if it has not yet been assigned a value (not even null
).
Check if a JavaScript variable is undefined
by using the typeof
operator, which will return the variable type as a string. You can then compare it using the equality operator '===
'.
var fruits;
console.log(typeof fruits === "undefined");
4. How to convert string to number
Convert a JavaScript string to an integer using parseInt()
. (Note that any fraction of the number will be removed.)
var myString = "3.14";
console.log(parseInt(myString));
Convert a JavaScript string to a decimal number using parseFloat()
.
var myString = "3.14";
console.log(parseFloat(myString));
Alternatively, you can use the Number()
function – returning either an integer or decimal (or NaN
– not a number) depending on the string.
var myString = "3.14";
console.log(Number(myString));
5. How to iterate through an object
Iterate through a JavaScript object using the for...in
statement.
var fruit = {
name: "Banana",
color: "Yellow",
texture: "Soft",
};
for (key in fruit) {
console.log(fruit[key]);
}
However, you need to be careful with this, as it will iterate over all enumerable properties which might not be something the object owns. So, depending on your use case, you might want to add a check for this using hasOwnProperty()
:
for (key in fruit) {
if (fruit.hasOwnProperty(key)) {
console.log(fruit[key]);
}
}
Also see the new Object.keys(), Object.values(), and Object.entries() methods that return an array of an object's keys, values and key-value pairs, respectively.
6. How to remove an item from an array
Use the pop()
method to remove the last item from a JavaScript array.
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.pop();
console.log(fruits);
Alternatively, you can use the splice()
method, indicating the position of the first element to be removed, followed by the number of elements to be removed. For example, to remove "Orange"
from the following array you would specify position 1
and 1
element, as follows:
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.splice(1, 1);
console.log(fruits);
Note that these solutions mutate the original array which goes against immutability practices. Refer to the note in How to add to an array above.
7. How to check if an element exists
To check if an element exists in the DOM using JavaScript, attempt to get the element, and if it does not exist, it will return null
.
For example, if you have an element with id "pitaya"
:
var elementExists = document.getElementById("pitaya") !== null;
console.log(elementExists);
8. How to redirect
Redirect to another URL in JavaScript using window.location.href
; for example:
window.location.href = "https://www.tiny.cloud/";
9. How to sleep
There are a lot of solutions out there that suggest using the combination of the Promise
object with the await
operator. However, they won’t work on IE11. So, where you need to support all browsers…
Implement sleep in JavaScript using setTimeout()
.
For example, here, the function passed into setTimeout()
will run 2 seconds after setTimeout()
is called.
console.log("Hey...");
setTimeout(function () {
console.log("...sorry, I forgot what I was going to say.");
}, 2000);
10. How to print an object
Print a JavaScript object with the JSON.stringify()
method.
var fruit = {
name: "Banana",
color: "Yellow",
texture: "Soft",
};
console.log(JSON.stringify(fruit));
BONUS TIP: How to add a rich text editor to your JavaScript project
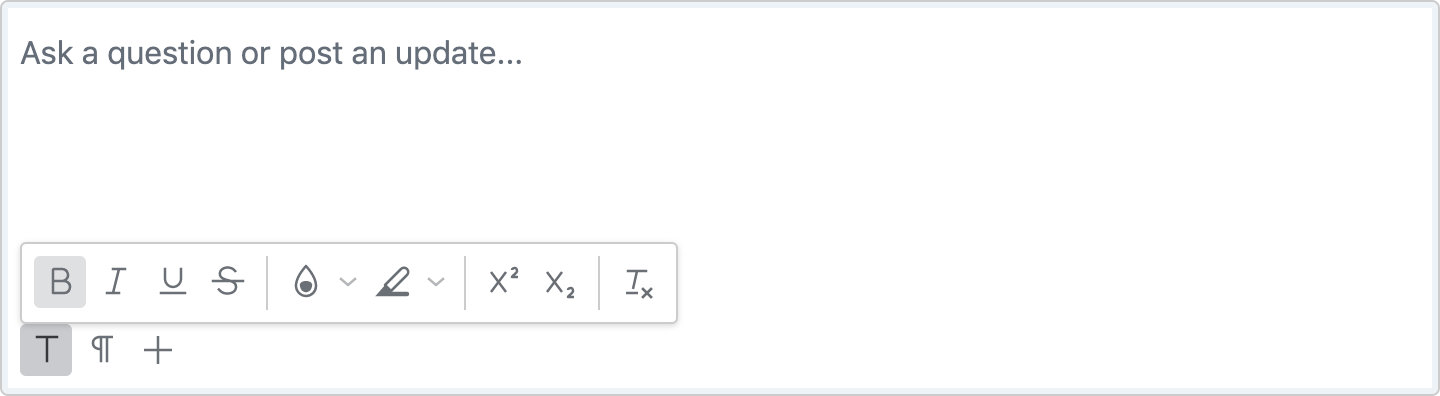
Okay, so it’s not in the top ten, but a lot of people ask us how to enhance their JavaScript apps with our open source rich text editor. Check out the following articles, depending on the particular framework you’re using:
- Enhance Bootstrap forms with WYSIWYG editing
- Enhance React forms with a rich text editor
- Create an Angular reactive form with a rich text editor
- Add a rich text editor to a simple Vue project
For more information about getting started with TinyMCE, check out how to get your free API key, plus our comprehensive guide to integrations. Or contact us to find out more about how other developers are taking advantage of rich text editing in their latest apps.
If this list of JavaScript how-tos has been helpful, share it with your friends and tag us @joinTiny. Also let us know if you have alternative solutions that you prefer.